python3 gtk3 update
This commit is contained in:
parent
4f04d13320
commit
dca5ef65ff
|
@ -1,6 +1,6 @@
|
|||
pyGtkRDP version 1.0
|
||||
pyGtkRDP version 1.3
|
||||
|
||||
Simple GUI application for freerdp or rdesktop connection
|
||||
Simple gtk3 application for freerdp or rdesktop connection.
|
||||
|
||||
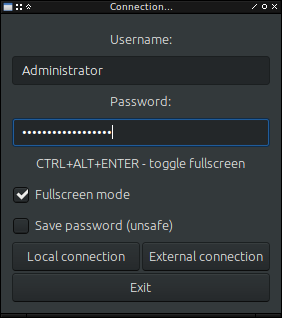
|
||||
|
||||
|
|
63
pygtkrdp.py
63
pygtkrdp.py
|
@ -1,12 +1,12 @@
|
|||
#!/usr/bin/env python
|
||||
#!/usr/bin/env python3
|
||||
# -*- coding: utf-8 -*-
|
||||
|
||||
import pygtk
|
||||
pygtk.require('2.0')
|
||||
import gtk
|
||||
import subprocess
|
||||
import base64
|
||||
import ConfigParser
|
||||
import subprocess
|
||||
import configparser
|
||||
import gi
|
||||
gi.require_version("Gtk", "3.0")
|
||||
from gi.repository import Gtk, Gdk, GLib
|
||||
from os import path
|
||||
|
||||
# ===== Connection settings: ======
|
||||
|
@ -21,7 +21,7 @@ string_r = "xfreerdp /drive:share,/media /bpp:8 /jpeg /cert-ignore /v:external.d
|
|||
|
||||
conf_file = path.expanduser("~") + '/.config/pygtkrdesktop.conf'
|
||||
|
||||
class Table:
|
||||
class Table(Gtk.Window):
|
||||
|
||||
def Connect(self, widget, data=None):
|
||||
name = login_entry.get_text()
|
||||
|
@ -50,7 +50,7 @@ class Table:
|
|||
rc = p.returncode
|
||||
|
||||
if ( (rc == 0) or (rc == 62)):
|
||||
gtk.main_quit()
|
||||
Gtk.main_quit()
|
||||
else:
|
||||
if (rc == 132):
|
||||
status_entry_label.set_markup('<b><span color="red">Error: wrong login or password</span></b>');
|
||||
|
@ -61,30 +61,31 @@ class Table:
|
|||
# print "Error code", rc
|
||||
|
||||
def LoginEnterPressed(self, widget, event):
|
||||
if gtk.gdk.keyval_name(event.keyval) == 'Return':
|
||||
if Gdk.keyval_name(event.keyval) == 'Return':
|
||||
password_entry.grab_focus()
|
||||
return True
|
||||
return False
|
||||
|
||||
def PasswordEnterPressed(self, widget, event):
|
||||
if gtk.gdk.keyval_name(event.keyval) == 'Return':
|
||||
if Gdk.keyval_name(event.keyval) == 'Return':
|
||||
self.Connect(widget, "local")
|
||||
return True
|
||||
return False
|
||||
|
||||
def delete_event(self, widget, event, data=None):
|
||||
gtk.main_quit()
|
||||
Gtk.main_quit()
|
||||
return False
|
||||
|
||||
def __init__(self):
|
||||
self.window = gtk.Window(gtk.WINDOW_TOPLEVEL)
|
||||
super().__init__(title="pyGtkRDP")
|
||||
self.window = Gtk.Window()
|
||||
self.window.set_title("Connection...")
|
||||
self.window.connect("delete_event", self.delete_event)
|
||||
self.window.set_border_width(10)
|
||||
table = gtk.Table(9, 2, True)
|
||||
table = Gtk.Table(n_rows=9, n_columns=2, homogeneous=True)
|
||||
self.window.add(table)
|
||||
|
||||
login_entry_label = gtk.Label ("Username:")
|
||||
login_entry_label = Gtk.Label (label="Username:")
|
||||
table.attach(login_entry_label, 0, 2, 0, 1)
|
||||
login_entry_label.show()
|
||||
|
||||
|
@ -95,7 +96,7 @@ class Table:
|
|||
login_entry.show()
|
||||
login_entry.connect('key-press-event', self.LoginEnterPressed)
|
||||
|
||||
password_entry_label = gtk.Label ("Password:")
|
||||
password_entry_label = Gtk.Label (label="Password:")
|
||||
table.attach(password_entry_label, 0, 2, 2, 3)
|
||||
password_entry_label.show()
|
||||
|
||||
|
@ -106,7 +107,8 @@ class Table:
|
|||
if conf.has_option("login", "saved"):
|
||||
password_entry.grab_focus()
|
||||
if conf.has_option("login", "password"):
|
||||
password_entry.set_text(base64.b32decode(base64.b16decode(base64.b64decode(conf.get("login", "password")))))
|
||||
password_entry.set_text(base64.b32decode(base64.b16decode(base64.b64decode
|
||||
(conf.get("login", "password")))).decode('utf-8'))
|
||||
password_entry.connect('key-press-event', self.PasswordEnterPressed)
|
||||
|
||||
table.attach(status_entry_label, 0, 2, 4, 5)
|
||||
|
@ -134,20 +136,20 @@ class Table:
|
|||
table.attach(password_check, 0, 2, 6, 7)
|
||||
password_check.show()
|
||||
|
||||
local_button = gtk.Button("Local connection")
|
||||
local_button = Gtk.Button(label="Local connection")
|
||||
local_button.set_tooltip_text("Connection via LAN (using internal IP.")
|
||||
local_button.connect("clicked", self.Connect, "local")
|
||||
table.attach(local_button, 0, 1, 7, 8)
|
||||
local_button.show()
|
||||
|
||||
remote_button = gtk.Button("External connection")
|
||||
remote_button = Gtk.Button(label="External connection")
|
||||
remote_button.set_tooltip_text("Connection via WAN (using external IP).")
|
||||
remote_button.connect("clicked", self.Connect, "remote")
|
||||
table.attach(remote_button, 1, 2, 7, 8)
|
||||
remote_button.show()
|
||||
|
||||
button = gtk.Button("Exit")
|
||||
button.connect("clicked", lambda w: gtk.main_quit())
|
||||
button = Gtk.Button(label="Exit")
|
||||
button.connect("clicked", lambda w: Gtk.main_quit())
|
||||
table.attach(button, 0, 2, 8, 9)
|
||||
button.show()
|
||||
|
||||
|
@ -161,24 +163,27 @@ def SaveLogin(login, password):
|
|||
conf.set("login", "fullscreen", fullscreen_check.get_active())
|
||||
conf.set("login", "savepassword", password_check.get_active())
|
||||
if password_check.get_active():
|
||||
conf.set("login", "password",
|
||||
base64.b64encode(base64.b16encode(base64.b32encode(password))))
|
||||
password = password.encode()
|
||||
password = base64.b64encode(base64.b16encode(
|
||||
base64.b32encode(password)))
|
||||
password = password.decode('utf-8')
|
||||
conf.set("login", "password", password)
|
||||
else:
|
||||
conf.remove_option("login", "password")
|
||||
conf.write(open(conf_file, "w"))
|
||||
|
||||
def main():
|
||||
gtk.main()
|
||||
Gtk.main()
|
||||
return 0
|
||||
|
||||
login_entry = gtk.Entry()
|
||||
password_entry = gtk.Entry()
|
||||
status_entry_label = gtk.Label ("CTRL+ALT+ENTER - toggle fullscreen")
|
||||
fullscreen_check = gtk.CheckButton ("Fullscreen mode")
|
||||
password_check = gtk.CheckButton ("Save password (unsafe)")
|
||||
login_entry = Gtk.Entry()
|
||||
password_entry = Gtk.Entry()
|
||||
status_entry_label = Gtk.Label (label="CTRL+ALT+ENTER - toggle fullscreen")
|
||||
fullscreen_check = Gtk.CheckButton (label="Fullscreen mode")
|
||||
password_check = Gtk.CheckButton (label="Save password (unsafe)")
|
||||
|
||||
if __name__ == "__main__":
|
||||
conf = ConfigParser.RawConfigParser()
|
||||
conf = configparser.RawConfigParser()
|
||||
if not path.exists(conf_file):
|
||||
open(conf_file, 'w')
|
||||
conf.read(conf_file)
|
||||
|
|
Loading…
Reference in New Issue